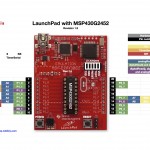
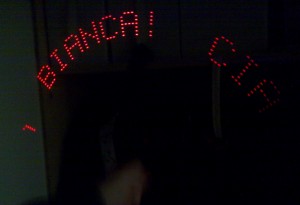
The LED POV shining in the dark.
Natale si avvicina ed è tempo di regali per i più piccini, ecco che con un po’ di materiale di recupero e una buona lente è possibile realizzare un simpatico gadget personalizzato che mostra una scritta nel buio.
Questo articolo è disponibile solo in inglese: puoi cambiare la lingua facendo clic sulla bandierina in alto.